1. 인스턴스(Instance) 멤버
◎ 인스턴스 멤버 : 필드와 메소드 등 객체(class)에 소속된 멤버
▷ 객체가 생성되어야만 사용 가능한 필드, 메소드입니다.
◎ this 키워드
▷ 객체 내부에서는 인스턴스 멤버에 접근하기 위해 this를 사용합니다. 객체는 자신을 'this'라고 지칭합니다.
▷ 생성자와 메소드의 매개변수명이 인스턴스 멤버인 필드명과 동일한 경우,
인스턴스 필드임을 강조하고자 할 때 this를 주로 사용합니다.
public class Car { // 필드 String model; int speed; Car(String model){ this.model = model; } // 메서드 void setSpeed(int speed) { this.speed = speed; } // 메서드 void run() { this.setSpeed(100); System.out.println(this.model + "가 달립니다.(시속:" + this.speed + "km/h)"); } }
인스턴스 멤버 : moded, speed, setSpeed(), run()public class CarExample { public static void main(String[] args) { Car myCar = new Car("포르쉐"); Car yourCar = new Car("벤츠"); myCar.run(); yourCar.run(); } } // 출력: // 포르쉐가 달립니다.(시속:100km/h) // 벤츠가 달립니다.(시속:100km/h)
2. 정적(Static) 멤버
◎ 정적 멤버 : 정적멤버 선언만으로도 바로 사용 가능한 필드, 메소드
▷ 객체를 생성하더라도 같은 번지를 지칭하게 됩니다.
▷ 메소드 영역의 클래스에 고정적으로 위치하는 멤버입니다.
◎ static 키워드를 추가해 정적 필드와 정적 메소드로 선업합니다.
◎ 정적 멤버 사용
▷ 클래스가 메모리로 로딩되면 정적 멤버를 바로 사용할 수 있습니다.
▷ 클래스 이름과 함께 도트(.) 연산자로 접근합니다.
public class Calculator { static double pi = 3.14159; static int plus(int x, int y) { return x + y; } static int minus(int x, int y) { return x - y; } }
public class CalculatorExample { public static void main(String[] args) { double result1 = 10 * 10 * Calculator.pi; double result2 = Calculator.plus(10, 5); double result3 = Calculator.minus(10, 5); System.out.println("result1 : " + result1); System.out.println("result2 : " + result2); System.out.println("result3 : " + result3); } } // 출력 : // result1 : 314.159 // result2 : 15.0 // result3 : 5.0
static의 장점은 별도로 객체를 하나 만들지 않아도 된다는 것입니다.
만약 instance 멤버를 사용했다면 별도의 객체를 만들었을 것입니다.
ex) Calculator myCalc = new Calculator();
◎ 정적 블록
▷ 정적 필드를 선언할 때 복잡한 초기화 작업이 필요하다면 정적 블록을 이용합니다.
▷ 정적 블록은 클래스가 메모리로 로딩될 때 자동으로 실행합니다.
▷ 정적 블록이 클래스 내부에 여러 개가 선언되어 있을 경우에는 선언된 순서대로 실행합니다.
▷ 정적 필드는 객체 생성 없이도 사용할 수 있기 때문에 생성자에서 초기화 작업을 하지 않습니다.
public class Television { static String company = "MyCompany"; static String model = "LCD"; static String info; static { info = company + "-" + model; } }
public class TelevisionExample { public static void main(String[] args) { System.out.println(Television.info); } } // 출력: // MyCompany-LCD
◎ 인스턴스 멤버를 사용할 수 없을 때
▷ 정적 메소드와 정적 블록은 내부에 인스턴스 필드, 인스턴스 메소드, this를 사용할 수 없습니다.
▷ 정적 메소드와 정적 블록에서 인스턴스 멤버를 사용하고 싶다면 객체를 먼저 생성하고 참조 변수로 접근합니다.
public class test { // 인스턴스 멤버와 메소드 선언 <- 객체가 생성이 되어야지만 메모리에 올라간다. int field1; void method1() { } // 정적 멤버와 메소드 선언 <- 클래스 파일 생성만으로도 정적 멤버와 메소드가 메모리에 올라가진다. static int field2; static void method2() { } static { // field1 = 10; // method1(); field2 = 10; method2(); } static void method3() { // this.field1 = 10; // this.method1(); field2 = 10; method2(); test obj = new test(); obj.field1 = 10; obj.method1(); } }
public class Car { // 인스턴스 필드 int speed; // 인스턴스 메소드 void run() { System.out.println(speed + "으로 달립니다."); } // 정적 메소드 static void simulate() { Car myCar = new Car(); myCar.speed = 200; myCar.run(); } public static void main(String[] args) { simulate(); // 정적 메소드 호출 Car myCar = new Car(); myCar.speed = 60; myCar.run(); } } // 출력 : // 200으로 달립니다. // 60으로 달립니다.
인스턴스 멤버와 정적 멤버를 잘 이용해야 class 끼리 연결하거나 새로운 메서드를 연결할 때 문제없이 연결할 수 있습니다. 여기서 인스턴스란 객체의 의미로 사용하는 인스턴스와는 다르니 주의하셔야해요!!
많은 분들의 피드백은 언제나 환영합니다! 많은 댓글 부탁드려요~~
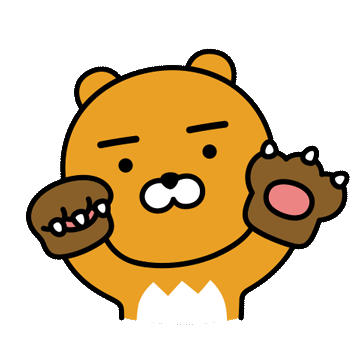
'BackEnd > Java' 카테고리의 다른 글
[java] 접근 제한자 (0) | 2023.01.11 |
---|---|
[java] 이것이 자바다 ch06 fianl 필드와 상수 (0) | 2023.01.11 |
[java] 이것이 자바다 ch06 메소드 (0) | 2023.01.11 |
[java] 이것이 자바다 ch06 클래스 (0) | 2023.01.11 |
[java] package jar 파일 만들기 (export/import) (0) | 2023.01.11 |