◎ 예제 1
public class Exception01 { public static void main(String[] args) { try { int[] a = {2, 0}; int b = 4; int c = b/a[2]; System.out.println(c); } catch(ArithmeticException e) { System.out.println("산술 오류 발생"); } catch(ArrayIndexOutOfBoundsException e) { System.out.println("배열 길이 오류발생"); } System.out.println("예외 처리 공부 중"); } } // 출력 : // 배열 길이 오류발생 // 예외 처리 공부 중
▷ a[2]를 사용해서 c에 저장했으므로 배열길이의 오류가 발생합니다.
▷ ArrayIndexOutOfBoundsException을 통해서 배열 길이 오류가 발생되면 catch할 수 있습니다.
◎ 예제 2
public class Exception02 { public static void main(String[] args) { int a = 0; int b = 2; try { System.out.println("외부로 접속"); int c = b/a; System.out.println("연결 해제"); } catch(ArithmeticException e) { System.out.println("오류 발생하였습니다."); } finally { System.out.println("무조건 연결 해제"); } } } // 출력 : // 외부로 접속 // 오류 발생하였습니다. // 무조건 연결 해제
▷ 2를 0으로 나누면 오류가 발생합니다.
▷ ArithmeticException은 0으로 나누면 무한대로 가기때문에 계산을 못하는 예외 발생시 catch하는 것입니다.
◎ 예제 3
public class Exception03 { public static void main(String[] args) { try { Exception e = new Exception("고의 예외"); throw e; } catch(Exception e) { System.out.println("예외 발생"); System.out.println(e.getMessage()); } } } // 출력 : // 예외 발생 // 고의 예외
▷ throw를 통해 e를 예외로 만들어주고 '예외 발생' 문구 먼저 출력합니다.
▷ 이후 getMessage를 통해 '고의 예외' 문구를 출력합니다.
◎ 예제 4
public class Exception04 { public static void methodA() throws Exception{ methodB(); } public static void methodB() throws Exception{ methodC(); } public static void methodC() throws Exception{ Exception e = new Exception(); throw e; } public static void main(String[] args) { try { methodA(); } catch(Exception e) { System.out.println("메인에서 처리"); } } } // 출력 : // 메인에서 처리
▷ methodC에서 에러를 Exception으로 보내고 methodB, methodA에서 차례로 호출하고 main에서 A를 호출합니다.
▷ 이때 예외가 있으면 catch에서 문구를 출력합니다.
◎ 예제 5
class AgeException extends Exception{ public AgeException() {} public AgeException(String message) { super(message); } } public class Exception05 { public static void ticketing(int age) throws AgeException{ if(age < 0){ throw new AgeException("나이 입력이 잘못되었습니다."); } } public static void main(String[] args) { int age = -19; try { ticketing(age); } catch(AgeException e) { e.printStackTrace(); } } } // 출력 : // java0118.AgeException: 나이 입력이 잘못되었습니다. // at java0118.Exception05.ticketing(Exception05.java:14) // at java0118.Exception05.main(Exception05.java:21)
▷ AgeException 클래스를 Exception의 자식 클래스로 만들어줍니다.
▷ age가 0보다 작으면 잘못입력되었다는 문구를 출력하고 AgeException으로 던져줍니다.
▷ 메인에서 age가 0보다 작기때문에 오류가 나타납니다.
◎ 예제 6
public class RethorwExample { /** Main method **/ public static void main(String[] args) { // Try some code try { System.out.println("외부 try..."); try { System.out.println("내부 try..."); Exception e = new Exception(); throw e; } catch(Exception e) { System.out.println("(내부 try-catch) exception : " + e); System.out.println("예외 던지기 한번 더 : "); throw e; } finally { System.out.println("finally 구문출력"); } } catch(Exception e) { System.out.println("(외부 try-catch)Catch exception : " + e); } System.out.println("종료"); } } // 출력 : // 외부 try... // 내부 try... // (내부 try-catch) exception : java.lang.Exception // 예외 던지기 한번 더 : // finally 구문출력 // (외부 try-catch)Catch exception : java.lang.Exception // 종료
▷ 먼저 외부 try를 실행하고 다음은 내부 try, throw를 통해 예외를 만들어주고 catch를 통해 예외를 받아 줍니다.
▷ catch문 안에 또 throw가 있기때문에 외부의 catch문에 예외를 받고 실행문을 출력합니다.
▷ 이때, 내부 catch문 다음에는 바로 finally가 실행한 후 외부 catch문을 실행합니다.
▷ 이후 종료 문구를 출력합니다.
이렇게 예외 처리를 다루는 예제들을 여러개 다뤄보았습니다!
좀 더 사용해보면서 익혀야겠어요!!
많은 분들의 피드백은 언제나 환영합니다! 많은 댓글 부탁드려요~~
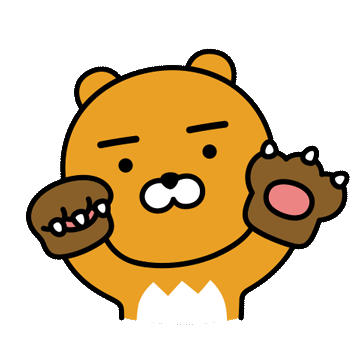
'BackEnd > Java' 카테고리의 다른 글
[java] 이것이 자바다 ch12 System 클래스 (0) | 2023.01.19 |
---|---|
[java] 이것이 자바다 ch12 java.base 모듈(Object 클래스) (0) | 2023.01.19 |
[java] ch11 예외 처리 2 (0) | 2023.01.19 |
[java] ch11 예외 처리 1 (0) | 2023.01.18 |
[java] 이것이 자바다 ch10 라이브러리와 모듈 (0) | 2023.01.17 |