728x90
반응형
1. Date 클래스
▷ 날짜를 표현하는 클래스로 객체 간에 날짜 정보를 주고받을 때 사용합니다.
▷ Date() 생성자는 컴퓨터의 현재 날짜를 읽어 Date 객체로 만듭니다.
Date now = new Date();
NO | 클래스 | 설명 |
1 | Date | 날짜 정보를 전달하기 위해 사용 |
2 | Calendar | 다양한 시간대별로 날짜와 시간을 얻을 때 사용 |
3 | LocalDateTime | 날짜와 시간을 조작할 때 사용 |
◎ Date 클래스 사용 예제
import java.text.*; import java.util.*; public class DateExample { public static void main(String[] args) { Date now = new Date(); String strNow1 = now.toString(); System.out.println(strNow1); SimpleDateFormat sdf = new SimpleDateFormat("yyyy.MM.dd HH:mm:ss"); String strNow2 = sdf.format(now); System.out.println(strNow2); } } // 출력 : // Thu Jan 19 16:52:57 KST 2023 // 2023.01.19 16:52:57
2. Calendar 클래스
▷ 달력을 표현하는 추상 클래스
▷ getInstance() 메소드로 컴퓨터에 설정된 시간대 기준으로 Calendar 하위 객체를 얻을 수 있음
Calendar now = Calendar.getInstance();
◎ 현재 시각 표시 예제
import java.util.*; public class CalendarExample { public static void main(String[] args) { Calendar now = Calendar.getInstance(); int year = now.get(Calendar.YEAR); int month = now.get(Calendar.MONTH) + 1; int day = now.get(Calendar.DAY_OF_MONTH); int week = now.get(Calendar.DAY_OF_WEEK); String strWeek = null; switch(week) { case Calendar.MONDAY: strWeek = "월"; break; case Calendar.TUESDAY: strWeek = "화"; break; case Calendar.WEDNESDAY: strWeek = "수"; break; case Calendar.THURSDAY: strWeek = "목"; break; case Calendar.FRIDAY: strWeek = "금"; break; case Calendar.SATURDAY: strWeek = "토"; break; default: strWeek = "일"; break; } int amPm = now.get(Calendar.AM_PM); String strAmPm = null; if(amPm == Calendar.AM) { strAmPm = "오전"; } else { strAmPm = "오후"; } int hour = now.get(Calendar.HOUR); int minute = now.get(Calendar.MINUTE); int second = now.get(Calendar.SECOND); System.out.print(year + "년 "); System.out.print(month + "월 "); System.out.println(day + "일 "); System.out.print(strWeek + "요일 "); System.out.println(strAmPm + " "); System.out.print(hour + "시 "); System.out.print(minute + "분 "); System.out.println(second + "초 "); } } // 출력 : // 2023년 1월 19일 // 목요일 오후 // 4시 54분 11초
◎ 지역 시간 출력
import java.util.Calendar; import java.util.TimeZone; public class LosAngelesExample { public static void main(String[] args) { TimeZone timeZone = TimeZone.getTimeZone("America/Los_Angeles"); Calendar now = Calendar.getInstance(timeZone); int amPm = now.get(Calendar.AM_PM); String strAmPm = null; if(amPm == Calendar.AM) { strAmPm = "오전"; } else { strAmPm = "오후"; } int hour = now.get(Calendar.HOUR); int minute = now.get(Calendar.MINUTE); int second = now.get(Calendar.SECOND); System.out.print(strAmPm + " "); System.out.print(hour + "시 "); System.out.print(minute + "분 "); System.out.println(second + "초 "); } } // 출력 : // 오전 0시 8분 11초
3. 날짜와 시간 조작
◎ 날짜 및 시간 변경 예제
import java.time.LocalDateTime; import java.time.format.DateTimeFormatter; public class DateTimeOperationExample { public static void main(String[] args) { LocalDateTime now = LocalDateTime.now(); DateTimeFormatter dtf = DateTimeFormatter.ofPattern("yyyy.MM.dd a HH:mm:ss"); System.out.println("현재 시간 : " + now.format(dtf)); LocalDateTime result1 = now.plusYears(1); System.out.println("1년 덧셈 : " + result1.format(dtf)); LocalDateTime result2 = now.minusMonths(2); System.out.println("2월 뺄셈 : " + result2.format(dtf)); LocalDateTime result3 = now.plusDays(7); System.out.println("7월 덧셈 : " + result3.format(dtf)); } } // 출력 : // 현재 시간 : 2023.01.19 오후 17:18:17 // 1년 덧셈 : 2024.01.19 오후 17:18:17 // 2월 뺄셈 : 2022.11.19 오후 17:18:17 // 7월 덧셈 : 2023.01.26 오후 17:18:17
4. 날짜와 시간 비교
▷ LocalDateTime 클래스는 날짜와 시간을 비교하는 메소드를 제공합니다.
NO | 리턴 타입 | 메소드(매개변수) | 설명 |
1 | boolean | isAfter(other) | 이후 날짜인지? |
2 | isBefore(other) | 이전 날짜인지? | |
3 | isEqual(other) | 동일 날짜인지? | |
4 | long | until(other, unit) | 주어진 단위(unit) 차이를 리턴 |
▷ 특정 날짜와 시간으로 LocalDateTime 객체를 얻는 방법
LocalDateTime target = LocalDateTime.of(year, month, dayOfMonth, hour, minute, second)
◎ 날짜 및 시간 비교 예제
import java.time.LocalDateTime; import java.time.format.DateTimeFormatter; import java.time.temporal.ChronoUnit; public class DateTimeCompareExample { public static void main(String[] args) { DateTimeFormatter dtf = DateTimeFormatter.ofPattern("yyyy.MM.dd a HH:mm:ss"); LocalDateTime startDateTime = LocalDateTime.now(); System.out.println("시작일 : " + startDateTime.format(dtf)); LocalDateTime endDateTime = LocalDateTime.of(2023,3,31,6,0,0); System.out.println("종료일 : " + endDateTime.format(dtf)); if(startDateTime.isBefore(endDateTime)) { //스타트 시간이 엔드 시간 이전에 있는지 확인해라 System.out.println("진행 중입니다."); } else if(startDateTime.isEqual(endDateTime)) { System.out.println("종료합니다."); } else if(startDateTime.isAfter(endDateTime)) { //스타트 시간이 엔드 시간 이후에 있는지 확인해라 System.out.println("종료합니다."); } long remainYear = startDateTime.until(endDateTime, ChronoUnit.YEARS); long remainMonth = startDateTime.until(endDateTime, ChronoUnit.MONTHS); long remainDay = startDateTime.until(endDateTime, ChronoUnit.DAYS); long remainHour = startDateTime.until(endDateTime, ChronoUnit.HOURS); long remainMinute = startDateTime.until(endDateTime, ChronoUnit.MINUTES); long remainSecond = startDateTime.until(endDateTime, ChronoUnit.SECONDS); System.out.println("남은 해 : " + remainYear); System.out.println("남은 월 : " + remainMonth); System.out.println("남은 일 : " + remainDay); System.out.println("남은 시간 : " + remainHour); System.out.println("남은 분: " + remainMinute); System.out.println("남은 초 : " + remainSecond); } } // 출력 : // 시작일 : 2023.01.19 오후 17:46:31 // 종료일 : 2023.03.31 오전 06:00:00 // 진행 중입니다. // 남은 해 : 0 // 남은 월 : 2 // 남은 일 : 70 // 남은 시간 : 1692 // 남은 분: 101533 // 남은 초 : 6092008
Date, Calendar 클래스를 이용한 예제들을 다뤄보았습니다.
이 클래스들은 익숙하지 않은 메소드들이 많이 나와서 아직 제대로 이해가 되지 않네요,,
많은 분들의 피드백은 언제나 환영합니다! 많은 댓글 부탁드려요~~
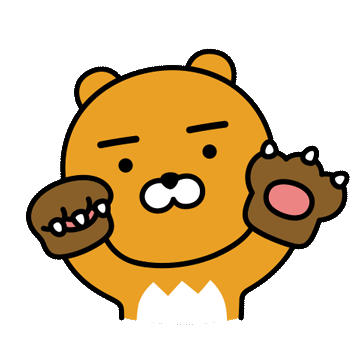
728x90
반응형
'BackEnd > Java' 카테고리의 다른 글
[java] 이것이 자바다 ch12 리플렉션(reflection) (0) | 2023.01.21 |
---|---|
[java] 이것이 자바다 ch12 정규 표현식 클래스 (0) | 2023.01.21 |
[java] 이것이 자바다 ch12 수학(Math) 클래스 (0) | 2023.01.19 |
[java] 이것이 자바다 ch12 포장 클래스 (0) | 2023.01.19 |
[java] 이것이 자바다 ch12 문자열 클래스 (0) | 2023.01.19 |