6. LIFO와 FIFO 컬렉션
◎ 후입 선출과 선입선출
▷ 후입 섭출(LIFO : Last In First Out) : 스택(stack)
▶ 나중에 넣은 객체가 먼저 빠져나가는 구조
▷ 선입 선출(FIFO : First In First Out) : 큐(queue)
▶ 먼저 넣은 객체가 먼저 빠져나가는 구조
▷ 컬렉션 프레임워크는 LIFO 자료구조를 제공하는 스택 클래스와 FIFO 자료 구조를 제공하는 큐 인터페이스 제공
◎ Stack
▷ Stack 클래스 : LIFO 자료구조를 구현한 클래스
NO | 리턴 타입 | 메소드 | 설명 |
1 | E | push(E item) | 주어진 객체를 스택에 넣는다. |
2 | E | pop() | 스택의 맨 위 객체를 빼낸다. |
◎ Stack 컬렉션 사용 예제
1. Coin 클래스
public class Coin { private int value; public Coin(int value) { this.value = value; } public int getValue() { return value; } }
2. StackExample 메인 클래스
import java.util.Stack; public class StackExample { public static void main(String[] args) { // Stack 컬렉션 생성 Stack<Coin> coinBox = new Stack<Coin>(); // 동전 넣기 coinBox.push(new Coin(100)); coinBox.push(new Coin(50)); coinBox.push(new Coin(500)); coinBox.push(new Coin(10)); // 동전을 하나씩 꺼내기 while(!coinBox.isEmpty()) { Coin coin = coinBox.pop(); System.out.println("꺼내온 동전 : " + coin.getValue() + "원"); } } } // 출력 : // 꺼내온 동전 : 10원 // 꺼내온 동전 : 500원 // 꺼내온 동전 : 50원 // 꺼내온 동전 : 100원
◎ Queue
▷ Queue 인터페이스 : FIFO 자료 구조에서 사용되는 메소드를 정의
▷ LinkedList : Queue 인터페이스를 구현한 대표적인 클래스
NO | 리턴 타입 | 메소드 | 설명 |
1 | boolean | offer(E e) | 주어진 객체를 큐에 넣는다. |
2 | E | poll() | 큐에서 객체를 빼낸다. |
◎ Queue 컬렉션 사용 예제
1. Message 클래스
public class Message { public String command; public String to; public Message(String command, String to) { this.command = command; this.to = to; } }
2. QueueExample 메인 클래스
import java.util.LinkedList; import java.util.Queue; public class QueueExample { public static void main(String[] args) { // Queue 컬렉션 생성 Queue<Message> messageQueue = new LinkedList<>(); // 메시지 넣기 messageQueue.offer(new Message("sendMail", "홍길동")); messageQueue.offer(new Message("sendSMS", "신용권")); messageQueue.offer(new Message("sendKakaotalk", "감자바")); // 메시지를 하나씩 꺼내어 처리 while(!messageQueue.isEmpty()) { Message message = messageQueue.poll(); switch(message.command) { case "sendMail": System.out.println(message.to + "님에게 메일을 보냅니다."); break; case "sendSMS": System.out.println(message.to + "님에게 SMS을 보냅니다."); break; case "sendKakaotalk": System.out.println(message.to + "님에게 카카오톡을 보냅니다."); break; } } } } // 출력 : // 홍길동님에게 메일을 보냅니다. // 신용권님에게 SMS을 보냅니다. // 감자바님에게 카카오톡을 보냅니다.
7. 동기화된 컬렉션
▷ 동기화된 메소드로 구성된 Vector와 Hashtable는 멀티스레드 환경에서 안전하게 요소를 처리
▷ Collections의 synchronizedXXX() 메소드 : ArrayList, HashSet, HashMap 등 비동기화된 메소드를 동기화된 메소드로 래핑
NO | 리턴 타입 | 메소드 | 설명 |
1 | List<T> | synchronizedList(List<T> list) | List를 동기화된 List로 리턴 |
2 | Map<K,V> | synchronizedMap(Map<K,V m) | Map을 동기화된 Map으로 리턴 |
3 | Set<T> | synchronizedSet(Set<T> s) | Set을 동기화된 Set으로 리턴 |
◎ 동기화된 컬렉션 예제
import java.util.Collections; import java.util.HashMap; import java.util.Map; public class SynchronizedMapExample { public static void main(String[] args) { // Map 컬렉션 생성 Map<Integer, String> map = Collections.synchronizedMap(new HashMap<>()); // 작업 스레드 객체 생성 Thread threadA = new Thread() { @Override public void run() { // 객체 1000개 추가 for(int i=1; i<=1000; i++) { map.put(i, "내용"+i); } } }; // 작업 스레드 객체 생성 Thread threadB = new Thread() { @Override public void run() { // 객체 1000개 추가 for(int i=1001; i<=2000; i++) { map.put(i, "내용"+i); } } }; // 작업 스레드 실행 threadA.start(); threadB.start(); // 작업 스레드들이 모두 종료될 때까지 메인 스레드를 기다리게 함 try { threadA.join(); threadB.join(); } catch(Exception e) { } // 저장된 총 객체 수 얻기 int size = map.size(); System.out.println("총 객체 수 : " + size); System.out.println(); } } // 출력 : // 총 객체 수 : 2000
8. 수정할 수 없는 컬렉션
▷ 요소를 추가, 삭제할 수 없는 컬렉션. 컬렉션 생성시 저장된 요소를 변경하고 싶지 않을 때 유용합니다.
▷ List, Set, Map 인터페이스의 정적 메소드인 of()로 생성
▷ List, Set, Map 인터페이스의 정적 메소드인 copyOf()을 이용해 기존 컬렉션을 복사
▷ 배열로부터 수정할 수 없는 List 컬렉션을 만듦.
◎ 수정할 수 없는 컬렉션 예제
import java.util.ArrayList; import java.util.Arrays; import java.util.HashMap; import java.util.HashSet; import java.util.List; import java.util.Map; import java.util.Set; public class ImmutableExample { public static void main(String[] args) { // List 불변 컬렉션 생성 List<String> immutableList1 = List.of("A", "B", "C"); // immutableList1.add("D"); (X) // Set 불변 컬렉션 생성 Set<String> immutableSet1 = Set.of("A", "B", "C"); // immutableSet.remove("A"); (X) Map<Integer, String> immutableMap1 = Map.of( 1, "A", 2, "B", 3, "C" ); // immutableMap1.put(4, "D"); (x) // List 컬렉션을 불변 컬렉션으로 복사 List<String> list = new ArrayList<>(); list.add("A"); list.add("B"); list.add("C"); List<String> immutableList2 = List.copyOf(list); // Set 컬렉션을 불변 컬렉션으로 복사 Set<String> set = new HashSet<>(); set.add("A"); set.add("B"); set.add("C"); Set<String> immutableSet2 = Set.copyOf(set); // Map 컬렉션을 불변 컬렉션으로 복사 Map<Integer, String> map = new HashMap<>(); map.put(1, "A"); map.put(2, "B"); map.put(3, "C"); Map<Integer, String> immutableMap2 = Map.copyOf(map); // 배열로부터 List 불변 컬렉션 생성 String[] arr = { "A", "B", "C" }; List<String> immutableList3 = Arrays.asList(arr); } }
▷ of를 사용하면 수정할 수 없습니다.
▷ 해당 코드는 java8에서는 사용할 수 없습니다.
컬렉션의 마지막 장까지 모두 마무리해보았습니다.
동기화와 Stack, Queue 등의 예제들도 모두 실행해보았는데요 컴퓨터 구조에 대해서 좀 더 자세히 알아봐야겠어요!
많은 분들의 피드백은 언제나 환영합니다! 많은 댓글 부탁드려요~~
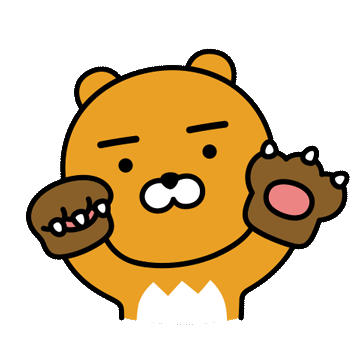
'BackEnd > Java' 카테고리의 다른 글
[java] 이것이 자바다 ch17 스트림(내부 반복자, 파이프라인, 인터페이스) (2) | 2023.01.27 |
---|---|
[java] 이것이 자바다 ch16 람다식(rambda) (0) | 2023.01.27 |
[java] 이것이 자바다 ch15 컬렉션 2 (0) | 2023.01.26 |
[java] 이것이 자바다 ch15 컬렉션 1 (0) | 2023.01.25 |
[java] 이것이 자바다 ch14 스레드(thread) 2 (0) | 2023.01.25 |