5. 요소 걸러내기(필터링)
▷ 필터링은 요소를 걸러내는 중간 처리 기능
◎ 필터링 메소드
NO | 리턴 타입 | 메소드(매개변수) | 설명 |
1 | Stream IntStream LongStream DoubleStream |
distinct() | 중복 제거 |
filter(Predicate<T>) filter(intPredicate) filter(LongPredicate) filter(DoublePredicate) |
조건 필터링 매개 타입은 요소 타입에 따른 함수형 인터페이스이므로 람다식으로 작성 가능 |
▷ distinct() 메소드 : 요소의 중복을 제거
▷ filter() 메소드 : 매개값으로 주어진 Predicate가 true를 리턴하는 요소만 필터링
▷ Predicae : 함수형 인터페이스
◎ 검사하는 추상 메소드
NO | 인터페이스 | 추상 메소드 | 설명 |
1 | Predicate<T> | boolean test(T t) | 객체 T를 조사 |
2 | IntPredicate | boolean test(int value) | int 값을 조사 |
3 | LongPredicate | boolean test(long value) | long 값을 조사 |
4 | DoublePredicate | boolean test(double value) | double 값을 조사 |
▷ 모든 Predicate는 매개값을 조사한 후 boolean을 리턴하는 test() 메소드를 가지고 있습니다.
◎ 필터링 예제
import java.util.ArrayList; import java.util.List; public class FilteringExample { public static void main(String[] args) { // List 컬렉션 생성 List<String> list = new ArrayList<>(); list.add("홍길동"); list.add("신용권"); list.add("감자바"); list.add("신용권"); list.add("신민철"); // 중복 요소 제거 list.stream() .distinct() .forEach(n -> System.out.println(n)); System.out.println(); // 신으로 시작하는 요소만 필터링 list.stream() .filter(n -> n.startsWith("신")) .forEach(n -> System.out.println(n)); System.out.println(); // 중복 요소를 먼저 제거하고, 신으로 시작하는 요소만 필터링 list.stream() .distinct() .filter(n -> n.startsWith("신")) .forEach(n -> System.out.println(n)); System.out.println(); } } // 출력 : // 홍길동 // 신용권 // 감자바 // 신민철 // // 신용권 // 신용권 // 신민철 // // 신용권 // 신민철
6. 요소 변환(매핑)
▷ 스트림의 요소를 다른 요소로 변환하는 중간 처리 기능
▷ 매핑 메소드 : mapXxx(), asDoubleStream(), asLongStream(), boxed(), flatMapXxx() 등
◎ 요소를 다른 요소로 변환
▷ mapXxx() 메소드 : 요소를 다른 요소로 변환한 새로운 스트림을 리턴
◎ 변환 메소드
NO | 리턴 타입 | 메소드(매개변수) | 요소 → 변환 요소 |
1 | Stream<R> | map(Function<T,R>) | T → R |
2 | IntStream LongStream DoubleStream |
mapToInt(ToIntFunction<T>) | T → int |
3 | mapToLong(ToLongFunction<T>) | T → long | |
4 | mapToDouble(ToDoubleFunction<T>) | T → double | |
5 | Stream<U> | mapToObj(intFunction<U>) | int → U |
6 | mapToObj(LongFunction<U>) | long → U | |
7 | mapToObj(DoubleFunction<U>) | double → U | |
8 | DoubleStream DoubleStream IntStream LongStream |
mapToDouble(IntToDoubleFunction) | int → double |
9 | mapToDouble(LongToDoubleFunction) | long → double | |
10 | mapToInt(DoubleToIntFunction) | double → int | |
11 | mapToLong(DoubleToLongFunction) | double → long |
▷ 매개타입인 Function은 함수형 인터페이스
◎ 변환 추상 메소드
NO | 인터페이스 | 추상 메소드 | 매개값 → 리턴값 |
1 | Function<T,R> | R apply(T t) | T → R |
2 | IntFunction<R> | R apply(int value) | int → R |
3 | LongFunction<R> | R apply(long value) | long → R |
4 | DoubleFunction<R> | R apply(double value) | double → R |
5 | ToIntFunction<T> | int applyAsInt(T value) | T → int |
6 | ToLongFunction<T> | long applyAsLong(T value) | T → long |
7 | ToDoubleFunction<T> | double applyAsDouble(T value) | T → double |
8 | IntToLongFunction | long applyAsLong(int value) | int → long |
9 | IntToDoubleFunction | double applyAsDouble(int value) | int → double |
10 | LongToIntFunction | int applyAsInt(long value) | long → int |
11 | LongToDoubleFunction | double applyAsDouble(long value) | long → double |
12 | DoubleToIntFunction | int applyAsInt(double value) | double → int |
13 | DoubleToLongFunction | long applyAsLong(double value) | double → long |
▷ 모든 Function은 매개값을 리턴값으로 매핑(변환)하는 applyXxx() 메소드를 가집니다.
◎ 매핑 예제
1. Student 클래스
public class Student { private String name; private int score; public Student(String name, int score) { this.name = name; this.score = score; } public String getName() { return name; } public int getScore() { return score; } }
2. MapExample 메인 클래스
import java.util.ArrayList; import java.util.List; public class MapExample { public static void main(String[] args) { // List 컬렉션 생성 List<Student> studentList = new ArrayList<>(); studentList.add(new Student("홍길동", 85)); studentList.add(new Student("홍길동", 92)); studentList.add(new Student("홍길동", 87)); // Student를 score 스트림으로 변환 // mapToInt로 하지 않아도 되지만 기본 형태가 String이기 때문에 // 숫자 계산을 위해서는 숫자형으로 변경하는 것이 중요합니다. studentList.stream() .mapToInt(s -> s.getScore()) .forEach(score -> System.out.println(score)); System.out.println(); studentList.stream() .map(n -> n.getName()) .forEach(name -> System.out.println(name)); } } // 출력 : // 85 // 92 // 87 // // 홍길동 // 홍길동 // 홍길동
▷ 기본 타입 간의 변환이거나 기본 타입 요소를 래퍼(Wrapper) 객체 요소로 변환하려면 간편화 메소드를 사용할 수 있습니다.
◎ Wrapper 메소드
NO | 리턴 타입 | 메소드(매개변수) | 설명 |
1 | LongStream | asLongStream() | int → long |
2 | DoubleStream | asDoubleStream() | int → double long → double |
3 | Stream<Integer> Stream<Long> Stream<Double> |
boxed() | int → Integer long → Long double → Double |
◎ Wrapper 사용 예제
import java.util.Arrays; import java.util.stream.IntStream; public class MapExample { public static void main(String[] args) { int[] intArray = {1, 2, 3, 4, 5}; IntStream intStream = Arrays.stream(intArray); intStream .asDoubleStream() .forEach(d -> System.out.println(d)); System.out.println(); intStream = Arrays.stream(intArray); intStream .boxed() .forEach(obj -> System.out.println(obj.intValue())); } } // 출력 : // 1.0 // 2.0 // 3.0 // 4.0 // 5.0 // // 1 // 2 // 3 // 4 // 5
◎ 요소를 복수 개의 요소로 변환
▷ flatMapXxx() 메소드 : 하나의 요소를 복수 개의 요소들로 변환한 새로운 스트림을 리턴
◎ 요소 변환 예제(flatMap() 메소드 사용)
import java.util.ArrayList; import java.util.Arrays; import java.util.List; public class FlatMappingExample { public static void main(String[] args) { // 문장 스트림을 단어 스트림으로 변환 List<String> list1 = new ArrayList<>(); list1.add("this is java"); list1.add("i am a best developer"); list1.stream() .flatMap(data -> Arrays.stream(data.split(" "))) .forEach(word -> System.out.println(word)); System.out.println(); // 문자열 숫자 목록 스트림을 숫자 스트림으로 변환 List<String> list2 = Arrays.asList("10, 20, 30", "40, 50"); list2.stream() .flatMapToInt(data -> { String[] strArr = data.split(","); int[] intArr = new int[strArr.length]; for(int i=0; i<strArr.length; i++) { intArr[i] = Integer.parseInt(strArr[i].trim()); } return Arrays.stream(intArr); }) .forEach(number -> System.out.println(number)); } } // 출력 : // this // is // java // i // am // a // best // developer // // 10 // 20 // 30 // 40 // 50
스트림의 필터링, 타입 변환 메소드에 대해서 알아보았습니다!
이해는 되지만 왜 사용하는지, 왜 다른 메서드들을 사용하는지 잘 모르겠네요,,,ㅎㅎ
역시 복습이 중요한가봐요!!
많은 분들의 피드백은 언제나 환영합니다! 많은 댓글 부탁드려요~~
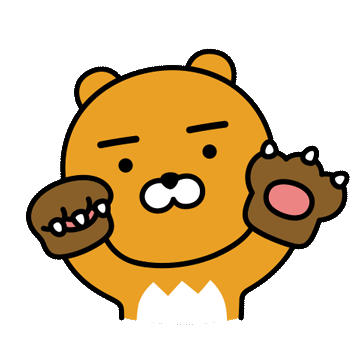
'BackEnd > Java' 카테고리의 다른 글
[java] 이것이 자바다 ch17 스트림(sorted, 루핑, 매칭, optional) (0) | 2023.01.30 |
---|---|
[백준 문제 10250번] ACM 호텔 문제 (0) | 2023.01.29 |
[java] 이것이 자바다 ch17 스트림(내부 반복자, 파이프라인, 인터페이스) (2) | 2023.01.27 |
[java] 이것이 자바다 ch16 람다식(rambda) (0) | 2023.01.27 |
[java] 이것이 자바다 ch15 컬렉션 3 (0) | 2023.01.26 |