https://bobo12.tistory.com/263
[JSP 웹 프로그래밍] JDBC 데이터베이스와 JSP 연동 2 (데이터베이스 쿼리 실행)
https://bobo12.tistory.com/261 [JSP 웹 프로그래밍] JDBC 데이터베이스와 JSP 연동 1 (JDBC 드라이버 로딩, DBMS 접속) 1. JDBC(Java DataBase Connectivity) ▷ 자바/JSP 프로그램 내에서 데이터베이스와 관련된 작업을 처
bobo12.tistory.com
4. ResultSet 객체
▷ Statement 또는 PreparedStatement 객체로 SELECT 문을 사용하여 얻어온 레코드 값을 테이블 형태로 가진 객체
◎ Statement 객체를 이용해 select 쿼리문 실행 결과 값 가져오기 예제
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@ page import="java.sql.*" %> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Database SQL</title> </head> <body> <%@ include file="dbconn.jsp" %> <table width="300" border="1"> <tr> <th>아이디</th> <th>비밀번호</th> <th>이름</th> </tr> <% ResultSet rs = null; Statement stmt = null; try{ String sql = "select * from member"; stmt = conn.createStatement(); rs = stmt.executeQuery(sql); while(rs.next()){ String id = rs.getString("id"); String pw = rs.getString("passwd"); String name = rs.getString("name"); %> <tr> <td><%=id %></td> <td><%=pw %></td> <td><%=name %></td> </tr> <% } } catch(SQLException ex){ out.println("Member 테이블 호출이 실패했습니다.<br>"); out.println("SQLException: " + ex.getMessage()); } finally { if(rs != null) rs.close(); if(stmt != null) stmt.close(); if(conn != null) conn.close(); } %> </table> </body> </html>
◎ PreparedStatement 객체를 이용해 select 쿼리문 실행 결과 값 가져오기 예제
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@ page import="java.sql.*" %> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Database SQL</title> </head> <body> <%@ include file="dbconn.jsp" %> <table width="300" border="1"> <tr> <th>아이디</th> <th>비밀번호</th> <th>이름</th> </tr> <% ResultSet rs = null; PreparedStatement pstmt = null; try{ String sql = "select * from member"; pstmt = conn.prepareStatement(sql); rs = pstmt.executeQuery(); while(rs.next()){ String id = rs.getString("id"); String pw = rs.getString("passwd"); String name = rs.getString("name"); %> <tr> <td><%=id %></td> <td><%=pw %></td> <td><%=name %></td> </tr> <% } } catch(SQLException ex){ out.println("Member 테이블 호출이 실패했습니다.<br>"); out.println("SQLException: " + ex.getMessage()); } finally { if(rs != null) rs.close(); if(pstmt != null) pstmt.close(); if(conn != null) conn.close(); } %> </table> </body> </html>
위의 예제와 동일한 결과 값이 출력됩니다.
◎ Statement 객체를 이용하여 update 쿼리문 실행 결과 값 가져오기 예제
1. update01.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Database SQL</title> </head> <body> <form method="post" action="update01_process.jsp"> <p>아이디 : <input type="text" name="id"> <p>비밀번호 : <input type="text" name="passwd"> <p>이름 : <input type="text" name="name"> <p><input type="submit" value="전송"> </form> </body> </html>
2. update01_process.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@ page import="java.sql.*" %> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Database SQL</title> </head> <body> <%@ include file="dbconn.jsp" %> <% request.setCharacterEncoding("utf-8"); String id = request.getParameter("id"); String passwd = request.getParameter("passwd"); String name = request.getParameter("name"); ResultSet rs = null; Statement stmt = null; try{ String sql = "select id, passwd from member where id='"+ id + "'"; stmt = conn.createStatement(); rs = stmt.executeQuery(sql); if(rs.next()){ String rId = rs.getString("id"); String rPasswd = rs.getString("passwd"); if(id.equals(rId) && passwd.equals(rPasswd)){ sql = "update member set name = '" + name + "' where id = '" + id + "'"; stmt = conn.createStatement(); stmt.executeUpdate(sql); out.println("Member 테이블을 수정했습니다."); } else out.println("일치하는 비밀번호가 아닙니다."); } else out.println("Member 테이블에 일치하는 아이디가 없습니다."); } catch (SQLException ex){ out.println("SQLEception: " + ex.getMessage()); } finally{ if(rs != null) rs.close(); if(stmt != null) stmt.close(); if(conn != null) conn.close(); } %> </body> </html>
위와 같이 변경하면 이름이 홍길순 → 관리자로 변경됩니다.
아이디와 패스워드가 일치하면 이름을 원하는 값으로 바꾸는 예제입니다.
◎ PaperedStatement 객체를 이용하여 update 쿼리문 실행 결과 값 가져오기 예제
1. update02.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Database SQL</title> </head> <body> <form method="post" action="update02_process.jsp"> <p>아이디 : <input type="text" name="id"> <p>비밀번호 : <input type="text" name="passwd"> <p>이름 : <input type="text" name="name"> <p><input type="submit" value="전송"> </form> </body> </html>
2. update02_process.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@ page import="java.sql.*" %> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Database SQL</title> </head> <body> <%@ include file="dbconn.jsp" %> <% request.setCharacterEncoding("utf-8"); String id = request.getParameter("id"); String passwd = request.getParameter("passwd"); String name = request.getParameter("name"); ResultSet rs = null; PreparedStatement pstmt = null; try{ String sql = "select id, passwd from member where id= ?"; pstmt = conn.prepareStatement(sql); pstmt.setString(1, id); rs = pstmt.executeQuery(); if(rs.next()){ String rId = rs.getString("id"); String rPasswd = rs.getString("passwd"); if(id.equals(rId) && passwd.equals(rPasswd)){ sql = "update member set name = ? where id = ?"; pstmt = conn.prepareStatement(sql); pstmt.setString(1, name); pstmt.setString(2, id); pstmt.executeUpdate(); out.println("Member 테이블을 수정했습니다."); } else out.println("일치하는 비밀번호가 아닙니다."); } else out.println("Member 테이블에 일치하는 아이디가 없습니다."); } catch (SQLException ex){ out.println("SQLEception: " + ex.getMessage()); } finally{ if(rs != null) rs.close(); if(pstmt != null) pstmt.close(); if(conn != null) conn.close(); } %> </body> </html>
위와 같이 변경하면 이름이 홍길동 → 수여인으로 변경됩니다.
pstmt를 통해 id와 일치하는 id, password 값들을 먼저 선택하고 그 값들이 일치하면 name을 수정할 수 있습니다.
여기서는 select문을 두 개 사용했네요!!
◎ Statement 객체를 이용하여 delete 쿼리문 실행 결과 값 가져오기 예제
1. delete01.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Database SQL</title> </head> <body> <form method="post" action="delete01_process.jsp"> <p>아이디 : <input type="text" name="id"> <p>비밀번호 : <input type="text" name="passwd"> <p><input type="submit" value="전송"> </form> </body> </html>
2. delete01_process.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@ page import="java.sql.*" %> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Database SQL</title> </head> <body> <%@ include file="dbconn.jsp" %> <% request.setCharacterEncoding("utf-8"); String id = request.getParameter("id"); String passwd = request.getParameter("passwd"); String name = request.getParameter("name"); ResultSet rs = null; Statement stmt = null; try{ String sql = "select id, passwd from member where id='"+ id + "'"; stmt = conn.createStatement(); rs = stmt.executeQuery(sql); if(rs.next()){ String rId = rs.getString("id"); String rPasswd = rs.getString("passwd"); if(id.equals(rId) && passwd.equals(rPasswd)){ sql = "delete from member where id = '" + id + "' and passwd = '" + passwd + "'"; stmt = conn.createStatement(); stmt.executeUpdate(sql); out.println("Member 테이블을 삭제했습니다."); } else out.println("일치하는 비밀번호가 아닙니다."); } else out.println("Member 테이블에 일치하는 아이디가 없습니다."); } catch (SQLException ex){ out.println("SQLEception: " + ex.getMessage()); } finally{ if(rs != null) rs.close(); if(stmt != null) stmt.close(); if(conn != null) conn.close(); } %> </body> </html>
id, passwd가 일치하면 해당 값이 삭제되는 예제입니다.
Statement 객체를 사용해서 delete문을 실행했습니다! 여기서도 selcet, delete를 둘 다 사용할 수 있네요!!
◎ PaperedStatement 객체를 이용하여 delete 쿼리문 실행 결과 값 가져오기 예제
1. delete02.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Database SQL</title> </head> <body> <form method="post" action="delete02_process.jsp"> <p>아이디 : <input type="text" name="id"> <p>비밀번호 : <input type="text" name="passwd"> <p><input type="submit" value="전송"> </form> </body> </html>
2. delete02_process.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@ page import="java.sql.*" %> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Database SQL</title> </head> <body> <%@ include file="dbconn.jsp" %> <% request.setCharacterEncoding("utf-8"); String id = request.getParameter("id"); String passwd = request.getParameter("passwd"); String name = request.getParameter("name"); ResultSet rs = null; PreparedStatement pstmt = null; try{ String sql = "select id, passwd from member where id= ?"; pstmt = conn.prepareStatement(sql); pstmt.setString(1, id); rs = pstmt.executeQuery(); if(rs.next()){ String rId = rs.getString("id"); String rPasswd = rs.getString("passwd"); if(id.equals(rId) && passwd.equals(rPasswd)){ sql = "delete from member where id = ? and passwd = ?"; pstmt = conn.prepareStatement(sql); pstmt.setString(1, id); pstmt.setString(2, passwd); pstmt.executeUpdate(); out.println("Member 테이블을 삭제했습니다."); } else out.println("일치하는 비밀번호가 아닙니다."); } else out.println("Member 테이블에 일치하는 아이디가 없습니다."); } catch (SQLException ex){ out.println("SQLEception: " + ex.getMessage()); } finally{ if(rs != null) rs.close(); if(pstmt != null) pstmt.close(); if(conn != null) conn.close(); } %> </body> </html>
id, passwd가 일치하면 삭제되는 예제입니다.
PreparedStatement 객체를 사용해서 delete문을 실행했습니다!
selcet, delete를 둘 다 사용했는데 Statement와 큰 차이점을 잘 모르겠네,,ㅎㅎ
ResultSet 객체는 select문을 사용해 얻어온 레코드 값을 테이블 형태로 가진 객체입니다.
테이블을 통해서 눈에 띄도록 구분해서 쿼리문들을 사용해봤습니다!!
사실 예제 해보면서 Statement와 PreparedStatement는 사용방법이 조금 다른 것 외에 어떤 점이 다른지 잘 모르겠네요,,
차이점을 한 번 찾아서 업데이트 하겠습니다!!
아는 분 있으시면 댓글로 남겨주세요~
많은 분들의 피드백은 언제나 환영합니다! 많은 댓글 부탁드려요~~
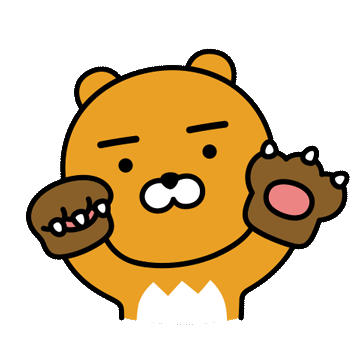
'BackEnd > JSP' 카테고리의 다른 글
[JSP 웹 프로그래밍] JSP 표준 태그 라이브러리 2 (sql 태그, function 태그) (0) | 2023.03.12 |
---|---|
[JSP 웹 프로그래밍] JSP 표준 태그 라이브러리 1 (Core 태그) (0) | 2023.03.12 |
[JSP 웹 프로그래밍] JDBC 데이터베이스와 JSP 연동 2 (데이터베이스 쿼리 실행) (1) | 2023.03.12 |
[JSP 웹 프로그래밍] JDBC 데이터베이스와 JSP 연동 1 (JDBC 드라이버 로딩, DBMS 접속) (1) | 2023.03.12 |
[JSP 웹 프로그래밍] 쿠키 2 (Cookie 정보 얻기, 삭제) (0) | 2023.03.12 |