◎ 다른 문제 풀이
[알고리즘] 정렬 문제 풀이(백준 10817번, 11399번)
◎ 다른 문제 풀이 [알고리즘] 정렬 문제 풀이(백준 2750번, 2751번, 10989번) (tistory.com) [알고리즘] 정렬 문제 풀이(백준 2750번, 2751번, 10989번) 1. 수 정렬하기(백준 2750번) 2750번: 수 정렬하기 (acmicpc.net)
bobo12.tistory.com
[알고리즘] 정렬 문제 풀이(백준 1181번, 1920번, 1427번)
1. 단어 정렬(백준 1181번 문제) 1181번: 단어 정렬 (acmicpc.net) 1181번: 단어 정렬 첫째 줄에 단어의 개수 N이 주어진다. (1 ≤ N ≤ 20,000) 둘째 줄부터 N개의 줄에 걸쳐 알파벳 소문자로 이루어진 단어가
bobo12.tistory.com
[알고리즘] 정렬 문제 풀이(백준 2750번, 2751번, 10989번)
◎ 다른 문제 풀이 [알고리즘] 정렬 문제 풀이(백준 10817번, 11399번) (tistory.com) [알고리즘] 정렬 문제 풀이(백준 10817번, 11399번) ◎ 다른 문제 풀이 [알고리즘] 정렬 문제 풀이(백준 2750번, 2751번, 10989
bobo12.tistory.com
1. 좌표 정렬하기(백준 11650번)
11650번: 좌표 정렬하기
첫째 줄에 점의 개수 N (1 ≤ N ≤ 100,000)이 주어진다. 둘째 줄부터 N개의 줄에는 i번점의 위치 xi와 yi가 주어진다. (-100,000 ≤ xi, yi ≤ 100,000) 좌표는 항상 정수이고, 위치가 같은 두 점은 없다.
www.acmicpc.net
▷ 풀이 코드
import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.util.Arrays; import java.util.Comparator; import java.util.Scanner; public class coordinateSort { public static void main(String args[]) throws IOException { BufferedReader br = new BufferedReader(new InputStreamReader(System.in)); StringBuilder sb = new StringBuilder(); int n = Integer.parseInt(br.readLine()); twoPoint point[] = new twoPoint[n]; for(int i=0; i<n; i++) { String[] input = br.readLine().split(" "); int x = Integer.parseInt(input[0]); int y = Integer.parseInt(input[1]); point[i] = new twoPoint(x, y); } Arrays.sort(point, new CoordinateComparator()); for(int i=0; i<n; i++) { sb.append(point[i].x).append(" ").append(point[i].y).append("\n"); } System.out.println(sb.toString()); } public static class twoPoint { int x; int y; public twoPoint(int x, int y) { this.x = x; this.y = y; } } public static class CoordinateComparator implements Comparator<twoPoint> { @Override public int compare(twoPoint p1, twoPoint p2) { if(p1.x != p2.x) { return p1.x - p2.x; } else{ return p1.y - p2.y; } } } }
2. 나이순 정렬(백준 10814번)
10814번: 나이순 정렬
온라인 저지에 가입한 사람들의 나이와 이름이 가입한 순서대로 주어진다. 이때, 회원들을 나이가 증가하는 순으로, 나이가 같으면 먼저 가입한 사람이 앞에 오는 순서로 정렬하는 프로그램을
www.acmicpc.net
▷ 풀이 코드
import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.util.Arrays; import java.util.Comparator; import java.util.Scanner; public class ageSort { public static void main(String args[]) throws IOException { BufferedReader br = new BufferedReader(new InputStreamReader(System.in)); StringBuilder sb = new StringBuilder(); int n = Integer.parseInt(br.readLine()); twoPoints points[] = new twoPoints[n]; for(int i=0; i<n; i++) { String temp[] = br.readLine().split(" "); int age = Integer.parseInt(temp[0]); String name = temp[1]; points[i] = new twoPoints(age, name); } Arrays.sort(points, new CoordinateComparator()); for(int i=0; i<n; i++) { sb.append(points[i].age).append(" ").append(points[i].name).append("\n"); } System.out.println(sb.toString()); } public static class twoPoints { int age; String name; public twoPoints(int age, String name) { this.age = age; this.name = name; } } public static class CoordinateComparator implements Comparator<twoPoints>{ @Override public int compare(twoPoints p1, twoPoints p2) { return p1.age - p2.age; } } }
이번에는 알고리즘 스터디를 통해 배운 정렬을 통해 백준 문제 풀이를 해보았습니다!!
sort에서 CoordinateComparator 클래스의 compare 함수를 Override하면 제가 원하는 대로 sort 함수를 사용할 수 있네요!!
다른 정렬 문제들도 풀어볼게요~
많은 분들의 피드백은 언제나 환영합니다! 많은 댓글 부탁드려요~~
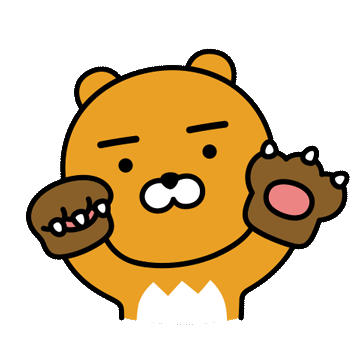
'문제풀기 > 알고리즘 스터디' 카테고리의 다른 글
[알고리즘] 정렬 문제 풀이(백준 2750번, 2751번, 10989번) (0) | 2023.06.07 |
---|---|
[알고리즘] 정렬 문제 풀이(백준 1181번, 1920번, 1427번) (0) | 2023.06.06 |
[알고리즘] 정렬 문제 풀이(백준 10817번, 11399번) (0) | 2023.06.05 |
[알고리즘] 재귀 문제 풀이(백준 27433번, 10870번, 25501번) (0) | 2023.05.14 |
[알고리즘] Queue 문제 풀이(백준 10773번, 10828번) (0) | 2023.05.07 |