1. textarea 태그
▷ 여러 줄의 텍스트를 입력할 수 있는 태그
▷ 기본 값은 <textarea>와 </textarea> 태그 사이에 설정
▷ 입력 폼 안에 사용된 태그와 띄어쓰기가 그대로 출력됨
◎ textarea 태그의 속성
◎ textarea를 이용한 가입 인사 추가 예제
<p> <textarea name = "comment" cols="30" rows="3" placeholder="가입 인사를 입력해주세요"></textarea>
출력 화면
2. 폼 데이터 처리하기
◎ 요청 파라미터의 값 받기
▷ request 내장 객체는 웹 브라우저가 서버로 보낸 요청에 대한 다양한 정보를 담고 있어 getParameter() 메소드를 이용하여 요청 파라미터의 값을 얻을 수 있음
◎ 체크 박스의 데이터를 전달받는 예제
1. index.jsp(데이터 전달)
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Form Processing</title> </head> <body> <form action = "process.jsp" method="POST"> <p> 독서<input type="checkbox" name="reading"> 운동<input type="checkbox" name="exercise"> 영화<input type="checkbox" name="movie"> <p> <input type="submit" value="전송"> </form> </body> </html>
2. process.jsp(데이터 전달 받음)
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Form Processing</title> </head> <body> <p> 독서 : <%= request.getParameter("reading") %> <p> 운동 : <%= request.getParameter("exercise") %> <p> 영화 : <%= request.getParameter("movie") %> </body> </html>
index.jsp 실행 후 원하는 값을 체크한 후 전송하면 아래와 같이 체크한 부분만 on 값이 나타납니다.
◎ 회원 가입 양식에서 폼 데이터 전송받기 예제 1
1. form04.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Form Processing</title> </head> <body> <h3>회원 가입</h3> <form action="form04_process.jsp" name="member" method="post"> <p> 아이디 : <input type="text" name="id"><input type="button" value="아이디 중복 검사"> <p> 비밀번호 : <input type="password" name="passwd"> <p> 이름 : <input type="text" name="name"> <p> 연락처 : <select name="phone1"> <option value="010">010</option> <option value="011">011</option> <option value="016">016</option> <option value="017">017</option> <option value="019">019</option> </select> - <input type="text" maxlength="4" size="4" name="phone2"> - <input type="text" maxlength="4" size="4" name="phone3"> <p> 성별 : <input type="radio" name="sex" value="남성" checked>남성 <input type="radio" name="sex" value="여성">여성 <p> 취미 : 독서<input type="checkbox" name="hobby1" checked> 운동<input type="checkbox" name="hobby2"> 영화<input type="checkbox" name="hobby3"> <p> <textarea name = "comment" cols="30" rows="3" placeholder="가입 인사를 입력해주세요"></textarea> <p><input type="submit" value="가입하기"> <input type="reset" value="다시 쓰기"> </form> </body> </html>
2. form04_process.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Form Processing</title> </head> <body> <% request.setCharacterEncoding("UTF-8"); String id = request.getParameter("id"); String passwd = request.getParameter("passwd"); String name = request.getParameter("name"); String phone1 = request.getParameter("phone1"); String phone2 = request.getParameter("phone2"); String phone3 = request.getParameter("phone3"); String sex = request.getParameter("sex"); String hobby1 = request.getParameter("hobby1"); String hobby2 = request.getParameter("hobby2"); String hobby3 = request.getParameter("hobby3"); String comment = request.getParameter("comment"); %> <p> 아이디 : <%= id %> <p> 비밀번호 : <%= passwd %> <p> 이름 : <%= name %> <p> 연락처 : <%= phone1 %> <%= phone2 %> <%= phone3 %> <p> 성별 : <%= sex %> <p> 취미 : <%= hobby1 %> <%= hobby2 %> <%= hobby3 %> <p> 가입 인사 : <%= comment %> </body> </html>
request를 통해 입력한 값들을 변수로 지정한 후 html의 body 부분에 작성해 결과를 출력할 수 있도록 합니다.
◎ 회원 가입 양식에서 폼 데이터 전송받기 예제 2
1. form05.jsp
... <form action="form05_process.jsp" name="member" method="post"> ... <p> 취미 : 독서<input type="checkbox" name="hobby" value="독서" checked> 운동<input type="checkbox" name="hobby" value="운동"> 영화<input type="checkbox" name="hobby" value="영화">
위의 form04.jsp에서 위의 코드를 수정 및 추가했습니다.
2. form05_process.jsp
... String[] hobby = request.getParameterValues("hobby"); ... <p> 취미 : <% if(hobby != null){ for(int i=0; i<hobby.length; i++){ out.println(" " + hobby[i]); } } %>
form04_process.jsp에서 hobby를 배열로 바꾼 후 for 문을 이용해서 문자로 값을 출력할 수 있습니다.
◎ 요청 파라미터의 전체 값 받기
▷ 요청 파라미터를 설정하지 않아도 모든 값을 전달받을 수 있음
▷ 텍스트 박스, 라디오 버튼, 드롭다운 박스와 같은 다양한 유형에 대해 한 번에 폼 데이터를 전달받을 수 있음
◎ 폼 데이터의 일괄 처리 메소드
◎ 체크 박스의 전체 데이터 전달받는 예제
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@ page import="java.io.*, java.util.*" %> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Form Processing</title> </head> <body> <table border="1"> <tr> <th>요청 파라미터 이름</th> <th>요청 파라미터 값</th> </tr> <% Enumeration paramNames = request.getParameterNames(); while(paramNames.hasMoreElements()){ String name = (String) paramNames.nextElement(); out.print("<tr><td>" + name + " </td>\n"); String paramValue = request.getParameter(name); out.println("<td> " + paramValue + "</td></tr>\n"); } %> </table> </body> </html>
◎ 회원 가입 양식에서 폼 데이터 전송받은 후 테이블에 적용 예제
1. form06.jsp
... <form action="form06_process.jsp" name="member" method="post"> ...
2. form06_process.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@ page import="java.io.*, java.util.*" %> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Form Processing</title> </head> <body> <table border="1"> <tr> <th>요청 파라미터 이름</th> <th>요청 파라미터 값</th> </tr> <% request.setCharacterEncoding("UTF-8"); Enumeration paramNames = request.getParameterNames(); while(paramNames.hasMoreElements()){ String name = (String) paramNames.nextElement(); out.print("<tr><td>" + name + " </td>\n"); String paramValue = request.getParameter(name); out.println("<td> " + paramValue + "</td></tr>\n"); } %> </table> </body> </html>
회원가입 페이지에서 아무것도 입력하지 않고 전송하면 아래와 같은 출력화면이 나타납니다.
textarea는 문자열을 입력할 수 있도록 만들어줍니다.
또 이번시간에는 폼의 데이터를 다른 jsp파일에 전달하고 한 번에 여러 데이터들을 전달하는 것까지 구현해보았습니다.
코드가 길어질수록 에러가 어디서 발생했는지 잘 모르겠네요,,,
에러가 발생한 위치를 잘 찾아서 수정하는 것을 목표로 연습해야겠어요!!
많은 분들의 피드백은 언제나 환영합니다! 많은 댓글 부탁드려요~~
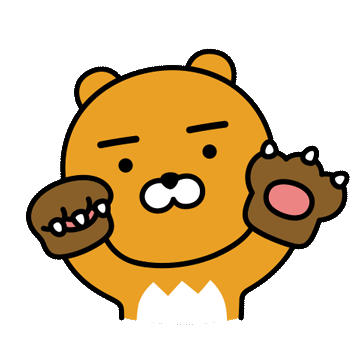
'BackEnd > JSP' 카테고리의 다른 글
[JSP 웹 프로그래밍] 유효성 검사 1 (validation) (0) | 2023.03.03 |
---|---|
[JSP 웹 프로그래밍] 파일 업로드 2 (Commons-FileUpload) (1) | 2023.03.02 |
[JSP 웹 프로그래밍] 폼 태그 1 (form, input, select) (0) | 2023.03.02 |
[JSP 웹 프로그래밍] 내장 객체 3 <javax> (out 내장 객체) (0) | 2023.03.01 |
[JSP 웹 프로그래밍] 내장 객체 2 <javax> (response) (0) | 2023.03.01 |