1.Thymeleaf 란
(1) 서버 사이드 렌더링
▷ 미리 정의된 템플릿(Template)을 만들고 동적으로 HTML 페이지를 만들어서 클라이언트에 전달하는 방식
▷ 요청이 올 때마다 서버에서 새로운 HTML 페이지를 만들어 주기 때문에 서버 사이드 렌더링 방식이라고 함
(2) Thymeleaf : 서버 사이드 템플릿 엔진의 한 종류
▷ Thymeleaf의 가장 큰 장점은 ‘natural templates’
▷ Thymeleaf를 사용할 때 Thymeleaf 문법을 포함하고 있는 html 파일을 서버 사이드 렌더링을 하지 않고
브라우저에 띄워도 정상적인 화면을 볼 수 있음
▷ 스프링에서 권장하는 서버 사이드 템플릿 엔진
◎ 웹 브라우저에서 Thymeleaf 파일 열어보기
1. 텍스트 파일로 아래와 같이 코드 작성하고 바탕화면에 저장
▷ thymeleafEx01.html로 파일 저장
▷ 저장된 아이콘 클릭시 홈페이지 화면 출력
◎ Thymeleaf 예제용 컨트롤러 클래스 생성
▷ src/main/java/com.shop.controller 패키지 생성 → ThymeleafExController 클래스 생성
1. ThymeleafExController 클래스 생성
package com.shop.controller; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RequestMapping; @Controller @RequestMapping(value = "/thymeleaf") public class ThymeleafExController { @GetMapping(value = "/ex01") public String thymeleafExample01(Model model){ model.addAttribute("data", "타임리프 예제입니다."); return "thymeleafEx/thymeleafEx01"; } }
2. src/main/java/templates → thymeleafEX 디렉토리 생성 → thymeleafEx01.html
<!DOCTYPE html> <html xmlns:th="http://www.thymeleaf.org"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> <p th:text="${data}">Hello Thymeleaf!!</p> </body> </html>
▷ ShopApplicationTests 실행한 후 아래의 경로로 접속시 출력되는 화면을 볼 수 있습니다.http://localhost:8080/thymeleaf/ex01
2. Spring Boot Devtools
▷ Spring Boot Devtools는 애플리케이션 개발 시 유용한 기능들을 제공하는 모듈
(1) Automatic Restart
▷ classpath에 있는 파일이 변경될 때마다 애플리케이션을 자동으로 재시작
(2) Live Reload
▷ 정적 자원(html, css, js) 수정 시 새로 고침 없이 바로 적용
(3) Property Defaults: Thymeleaf
▷ 기본적으로 성능을 향상시키기 위해서 캐싱 기능을 사용. 개발하는 과정에서 캐싱 기능을 사용한다면
수정한 소스가 제대로 반영되지 않을 수 있기 때문에 cache의 기본값을 false로 설정가능
◎ pom.xml에 의존성 추가
<!-- devtools 의존성 추가 --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> </dependency>
(1) Automatic Restart 적용
1. file → setting → Build, Execution, Deployment → Compiler 메뉴 선택 및 "Build Project automatically" 체크
2. file → setting → Advanced Settings → 아래의 문구 체크
브라우저의 새로고침을 하지 않아도 변경된 리소스가 웹 브라우저에 반영됨
(2) Live Reload 적용하기
◎ application.properties 내용 추가
#Live Reload 기능 활성화 spring.devtools.liverelodad.enabled=true
https://chrome.google.com/webstore/detail/livereload/jnihajbhpnppcggbcgedagnkighmdlei?hl=ko
위의 경로의 LiveReload 확장자 추가
◎ application.properties 내용 추가
▷ Thymeleaf의 캐싱 기능을 false 설정
▷ application.properties 분리 후 운영환경에서는 캐싱 기능을 사용하고,
개발환경에서는 캐싱 기능을꺼두는 방법으로 관리
#Thymeleaf cache 사용 중지 spring.thymeleaf.cache = false
(3) Thymeleaf 예제 진행
◎ th:text 예제
1. scr/main/java/com.shop.dto 패키지 생성 → ItemDto 클래스 생성
package com.shop.dto; import lombok.Getter; import lombok.Setter; import java.time.LocalDateTime; @Getter @Setter public class ItemDto { private Long id; private String itemNm; private Integer price; private String itemDetail; private String sellStatCd; private LocalDateTime regTime; private LocalDateTime updateTime; }
2. scr/main/java/com.shop.controller → ThymeleafExContorller 클래스 수정import com.shop.dto.ItemDto; ...(생략)... @GetMapping(value = "/ex02") public String thymeleafExample02(Model model){ ItemDto itemDto = new ItemDto(); itemDto.setItemDetail("상품 상세 설명"); itemDto.setItemNm("테스트 상품1"); itemDto.setPrice(10000); itemDto.setRegTime(LocalDateTime.now()); model.addAttribute("itemDto", itemDto); return "thymeleafEx/thymeleafEx02"; } }
3. src/main/java/templates.thymeleafEX → thymeleafEx02.html 생성
<!DOCTYPE html> <html xmlns:th="http://www.thymeleaf.org"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> <h1>상품 데이터 출력 예제</h1> <div> 상품명 : <span th:text="${itemDto.itemNm}"></span> </div> <div> 상품상세설명 : <span th:text="${itemDto.itemDetail}"></span> </div> <div> 상품등록일 : <span th:text="${itemDto.regTime}"></span> </div> <div> 상품가격 : <span th:text="${itemDto.price}"></span> </div> </body> </html>
http://localhost:8080/thymeleaf/ex02
위의 경로로 접속하면 아래와 같이 HTML이 적용된 화면이 출력되는 것을 확인할 수 있습니다.
Thymeleaf는 서버 사이드 템플릿 엔진의 한 종류로 미리 정의된 템플릿을 만들고 동적으로 HTML 페이지를 만들어 클라이언트에 전달합니다.
Controller와 html을 생성해 화면에 출력할 수 있도록 만들었습니다.
spring boot devtools는 애플리케이션 개발 시 유용한 기능들을 제공하는 모듈이며 개발환경에서는 캐싱 기능을 꺼둡니다.
th:text를 통해 TEST에 저장했던 값들을 html에 저장해서 경로를 지정하면 해당 값들을 출력할 수 있었습니다.
다른 문법들도 한 번 공부해볼게요~!!
많은 분들의 피드백은 언제나 환영합니다! 많은 댓글 부탁드려요~~
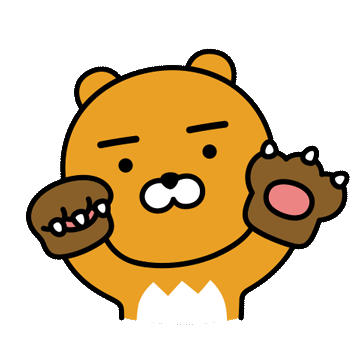