https://bobo12.tistory.com/324
7. 댓글 페이지의 화면 처리
▷ 게시물을 조회하는 페이지에 들어오면 기본적으로 가장 오래된 댓글들을 가져와서 1페이지에 보여줌
▷ 1페이지의 게시물을 가져올 때 해당 게시물의 댓글의 숫자를 파악해서 댓글의 페이지 번호를 출력함
▷ 댓글이 추가되면 댓글의 숫자만을 가져와서 최종 페이지를 찾아서 이동함
▷ 댓글의 수정과 삭제 후에는 다시 동일 페이지를 호출함
(1) 댓글 페이지 계산과 출력
▷ Ajax로 가져오는 데이터가 replyCnt와 list라는 데이터로 구성되므로 이를 처리하는 reply.js의 내용 역시 이를 처리하는 구조로 수정함
◎ reply.js 내용 수정
... (생략) ... function getList(param, callback, error) { var bno = param.bno; var page = param.page || 1; $.getJSON("/replies/pages/" + bno + "/" + page + ".json", function(data) { if(callback) { // callback(data); // 댓글 목록만 가져오는 경우 callback(data.replyCnt, data.list); // 댓글 숫자와 목록을 가져오는 경우 } }).fail(function(xhr, status, err) { if(error) { error(); } }); } ... (생략) ...
▷ 기존에 비해서 변경되는 부분은 callback 함수에 해당 게시물의 댓글 수(replyCnt)와 페이지에 해당하는 댓글 데이터를 전달하도록 하는 부분, 화면에 대한 처리는 get.jsp 내에서 이루어짐
◎ get.jsp 내용 수정
▷ reply.js를 이용해서 댓글의 페이지를 호출하는 부분은 showList 함수이므로 페이지 번호를 출력하도록 수정함
... (생략) ... function showList(page) { console.log("show list " + page); replyService.getList({bno:bnoValue, page:page||1}, function(replyCnt, list) { console.log("replyCnt: " + replyCnt); console.log("list: " + list); console.log(list); if(page == -1){ pageNum = Math.ceil(replyCnt/10.0); showList(pageNum); return; } var str = ""; if(list == null || list.length == 0) { replyUL.html(""); return; } for (var i = 0, len = list.length || 0; i<len; i++) { str +="<li class='left clearfix' data-rno='"+list[i].rno+"'>"; str +=" <div><div class='header'><strong class='primary-font'>["+list[i].rno+"] "+list[i].replyer+"</strong>"; str +=" <small class='pull-right text-muted'>"+replyService.displayTime(list[i].replyDate)+"</small></div>"; str +=" <p>"+list[i].reply+"</p></div></li>"; } replyUL.html(str); }); // end function } // end showList ... (생략) ...
▷ showList() 함수는 파라미터로 전달되는 page 변수를 이용해서 원하는 댓글 페이지를 가져오게 됨
▷ 이때 만일 page 번호가 -1로 전달되면 마지막 페이지를 찾아서 다시 호출함
▷ 사용자가 새로운 댓글을 추가하면 showList(-1); 을 호출하여 우선 전체 댓글의 숫자를 파악함
▷ 이 후에 다시 마지막 페이지를 호출해서 이동시키는 방식으로 동작시킴
이러한 방식은 여러번 서버를 호출해야하는 단점, 댓글의 등록 행위가 댓글 조회나 페이징에 비해서 적기 때문에 심각한 문제는 아닙니다.
◎ get.jsp... (생략) ... modalRegisterBtn.on("click", function(e){ var reply= { reply: modalInputReply.val(), replyer:modalInputReplyer.val(), bno:bnoValue }; replyService.add(reply, function(result){ alert(result); modal.find("input").val(""); modal.modal("hide"); // showList(1); showList(-1); }); }); ... (생략) ...
댓글은 화면상에서 댓글이 출력되는 영역의 아래쪽에 <div class='panel-footer'>를 하나 추가하고 <div>의 아래쪽에 추가.
◎ get.jsp 내용 수정
... (생략) ... <!-- /.panel-heading --> <div class="panel-body"> <ul class="chat"> ... (생략) ... </ul> <!-- /. end ul --> </div> <!-- /.panel .chat-panel --> <div class="panel-footer"> </div> </div> </div> <!-- ./ end row --> </div>
추가된 <div class='panel-footer'>에 댓글 페이지 번호를 출력하는 로직은 showReplyPage()는 아래와 같음
◎ get.jsp 내용 수정
... (생략) ... }); // end function } // end showList var pageNum = 1; var replyPageFooter = $(".panel-footer"); function showReplyPage(replyCnt) { var endNum = Math.ceil(pageNum / 10.0) * 10; var startNum = endNum - 9; var prev = startNum != 1; var next = false; if(endNum * 10 >= replyCnt) { endNum = Math.ceil(replyCnt/10.0); } if(endNum * 10 < replyCnt){ next = true; } var str = "<ul class='pagination pull-right'>"; if(prev){ str+= "<li class='page-item'><a class='page-link' href='"+(starNum -1)+"'>Previous</a></li>"; } for(var i = startNum; i<= endNum; i++){ var active = pageNum == i? "active":""; str+= "<li class='page-item "+active+" '><a class='page-link' href='"+i+"'>"+i"</a></li>"; } if(next){ str+="<li class='page-item'><a class='page-link' href='"+(endNum + 1)+"'>Next</a></li>"; } str += "</ul></div>"; console.log(str); replyPageFooter.html(str); } ... (생략) ...
▷ showReplyPage( ) 는 기존에 Java로 작성되는 PageMaker의 JavaScript 버전에 해당함
▷ 댓글 페이지를 문자열로 구성한 후 <div>의 innerHTML로 추가함
▷ showList()의 마지막에 페이지를 출력하도록 수정함// get.jsp ... 생략 ... replyUL.html(str); showReplyPage(replyCnt); }); // end function } // end showList ... 생략 ...
실제 화면상에는 CSS의 구성으로 인해 아래쪽에 추가
◎ 페이지 번호를 클릭했을 때 새로운 댓글을 가져오도록 get.jsp 내용 수정
... (생략) ... replyPageFooter.on("click","li a", function(e){ e.preventDefault(); console.log("page click"); var targetPageNum = $(this).attr("href"); console.log("targetPageNum: " + targetPageNum); pageNum = targetPageNum; showList(pageNum); }); ... (생략) ...
댓글의 페이지 번호는 <a> 태그 내에 존재하므로 이벤트 처리에서는 <a> 태그의 기본동작을 제한하고(preventDefault()) 댓글 페이지 번호를 변경한 후 해당 페이지의 댓글을 가져오도록 함
(2) 댓글의 수정과 삭제
▷ 댓글이 페이지 처리되면 댓글의 수정과 삭제 시에도 현재 댓글이 포함된 페이지로 이동하도록 수정함.
◎ get.jsp 내용 추가
... (생략) ... modalModBtn.on("click", function(e){ var reply = {rno:modal.data("rno"), reply: modalInputReply.val()}; replyService.update(reply, function(result){ alert(result); modal.modal("hide"); showList(pageNum); }); }); modalRemoveBtn.on("click", function (e) { var rno = modal.data("rno"); replyService.remove(rno, function(result) { alert(result); modal.modal("hide"); showList(pageNum); }); }); ... (생략) ...
기존과 달라진 점은 showList() 를 호출 할 때 현재 보고 있는 댓글 페이지의 번호를 호출 한다는 점입니다.
브라우저에서 댓글의 등록, 수정, 삭제 작업은 모두 페이지 이동을 하게 됩니다.
댓글 페이지의 화면 처리를 구현해 보았습니다. 오래된 댓글들을 1페이지에 보여주고 해당 페이지의 게시물을 가져올 때 해당 게시물의 댓글의 숫자를 파악해서 댓글의 페이지 번호를 출력하는 등 여러가지 기능들을 구현해보았습니다.
이렇게 스프링 공부를 해보았지만 아직 MVC에 대해서 자세히 이해하지를 못해서 그런지 확실하게 이해되는 것은 없네요,,
처음부터 책을 다시 한 번 읽어보고 따라해봐야 겠어요!!
다음은 스프링 부트에 대해서 공부해볼게요!!
많은 분들의 피드백은 언제나 환영합니다! 많은 댓글 부탁드려요~~
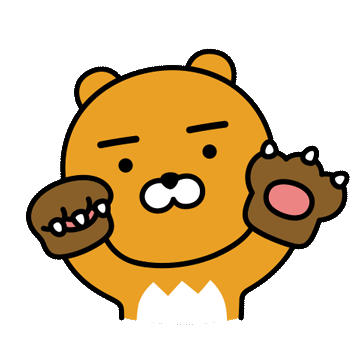
'BackEnd > Spring' 카테고리의 다른 글
[코드로 배우는 스프링 웹 프로젝트] ch17 Ajax 댓글 처리 7(댓글의 페이징 처리) (0) | 2023.04.03 |
---|---|
[코드로 배우는 스프링 웹 프로젝트] ch17 Ajax 댓글 처리 6 (이벤트 처리, HTML 처리) (0) | 2023.04.03 |
[코드로 배우는 스프링 웹 프로젝트] ch17 Ajax 댓글 처리 5 (댓글의 목록, 삭제 및 갱신, 수정, 조회 처리) (0) | 2023.04.03 |
[코드로 배우는 스프링 웹 프로젝트] ch17 Ajax 댓글 처리 4 (JavaScript의 모듈화) (0) | 2023.04.02 |
[코드로 배우는 스프링 웹 프로젝트] ch17 Ajax 댓글 처리 3 (ReplyController를 이용한 CRUD) (0) | 2023.04.02 |